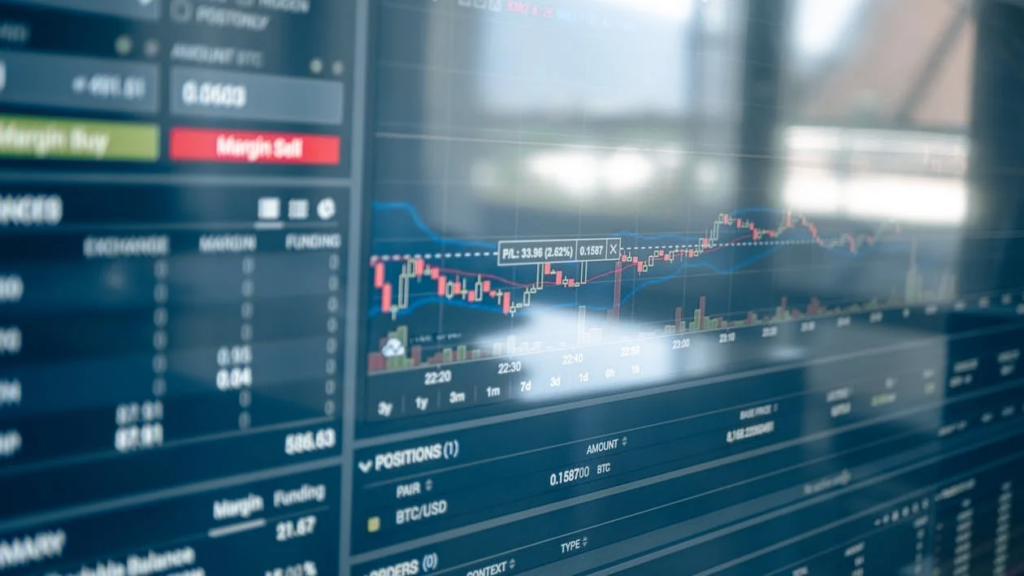
Introduction
In my last blog post Programmatically retrieving blockchain data – Part 1, I share a thorough guide on accessing and analyzing blockchain data programmatically. I emphasize the significance and advantages of this approach, highlighting benefits like real-time insights, transparency, trust, tailored analysis, automated auditing, smart contract oversight, innovation, research, and risk control. I walk readers through a detailed process of extracting transaction data from BSC Mainnet. This includes steps like registering with an RPC provider (where I particularly suggest QuickNode), procuring a BSC Mainnet Endpoint, fetching the most recent block number, sifting through that block for transactions, and then delving deep into the transaction details. Throughout the article, I’ve incorporated specific RPC methods and coding illustrations to facilitate a deeper grasp and practical application for my readers.
In this post I will walk through how to automate the process of getting transaction details using Node.js, a common library called web3, and using QuickNode as an RPC Provider.
Exercise
In this blog post, we’ll delve into a Node.js script that uses Web3 to fetch and display transaction details from a specific Ethereum-like blockchain.
Web3 Node.js Library
The web3.js library in Node.js is a powerful tool designed to facilitate interactions with the Ethereum blockchain. It enables developers to connect to local or remote Ethereum nodes, send transactions, deploy and interface with smart contracts, manage Ethereum accounts, and access blockchain data. With its modular design, web3.js abstracts the complexities of Ethereum, providing a streamlined JavaScript API for building decentralized applications and systems on the Ethereum network.
Source Code
Understanding the code
Let’s break down the provided code step by step:
1) Shebang Line
#!/usr/bin/node
This line specifies the interpreter for the script. It indicates that the script should be run using the Node.js runtime.
2) Importing Web3
const { Web3 } = require('web3');
The code imports the Web3
class from the ‘web3’ package, allowing us to interact with Ethereum-compatible blockchains.
3) Blockchain Node URL
var quicknodeurl = 'YOUR_QUICKNODE_URL';
This variable holds the URL of a blockchain node. In this case, it’s a QuickNode URL, which serves as the connection point to the blockchain network.
4) Provider URL Setup
const providerUrl = quicknodeurl;
const web3 = new Web3(providerUrl);
Here, the providerUrl
is set to the previously defined QuickNode URL, and a new instance of the Web3
class is created using this provider URL. This instance enables interaction with the blockchain.
5) Fetching Transaction Details
async function getTransactionDetails(transactionHash) {
// ...
}
This function takes a transaction hash as a parameter and asynchronously fetches transaction details using the web3.eth.getTransaction()
method. It then logs the retrieved transaction details to the console.
6) Fetching Transactions in a Block
async function getBlockTransactions(blockNumber) {
// ...
}
This function takes a block number as input and fetches details about transactions within that block using the web3.eth.getBlock()
method. It logs the block number and associated transaction hashes to the console. It also iterates through the transaction hashes and fetches details for each transaction using the getTransactionDetails()
function.
7) Fetching All Blocks
async function fetchAllBlocks() {
// ...
}
This function fetches the latest block number using web3.eth.getBlockNumber()
and iterates through blocks from the latest to the genesis block (block number 0). For each block, it calls the getBlockTransactions()
function to retrieve and display transaction details.
8) Executing the Fetching Process
fetchAllBlocks();
Finally, the fetchAllBlocks()
function is called to initiate the process of fetching and displaying transaction details for all blocks.
Conclusion
In this blog post, we explored a Node.js script that interacts with an Ethereum-compatible blockchain using the Web3 library. The script fetches transaction details and logs them to the console, providing insights into the blockchain’s transaction history. This code can be a useful starting point for anyone looking to programmatically explore and analyze blockchain data. Whether you’re a developer, researcher, or blockchain enthusiast, this example demonstrates how to harness the power of Web3 and Node.js for blockchain interaction.